Programming ESP8266 with GCC
Mikhail Grigorev has prepared Unofficial Development Kit for Espressif ESP8266 based on GCC for Xtensa core and SDK from Espressif. While recommended setup with Eclipse seems quite heavy to me same SDK can be used with Code::Blocks IDE.
Setup:
- install Code::Blocks (bundled with MinGW) if not installed
- install SDK into default (C:\Espressif) directory (paths inside makefiles are absolute)
- unpack coreutils.7z, ~300kB (additional tools required: mkdir.exe, mv.exe, rm.exe, true.exe) directory to SDK directory; these are taken from WinAVR
- from this point example projects would be (*) compilable from command line assuming that you have make tool
and coreutils directory would be added to PATH search list (**)
SET PATH=%PATH%;C:\Espressif\coreutils D:\progs\CodeBlocks\MinGW\bin\mingw32-make
* after changing mkdir to "mkdir.exe" (quotes are important!) inside Makefile to resolve conflict with internal shell command; otherwise you would see:The syntax of the command is incorrect.
To debug any other issues with make system try:D:\progs\CodeBlocks\MinGW\bin\mingw32-make VERBOSE=1
** adding to system PATH would not be necessary with Code::Blocks - either configure Code::Blocks manually (copy regular GCC settings creating new compiler configuration,
modify as shown below and add "C:\Espressif\coreutils" in "Additional Paths") or locate its configuration file
(with Win7: C:\Users\{username}\AppData\Roaming\CodeBlocks\default.conf)
and insert into it this compiler config snippet next to other compilers configurations;
Code::Blocks configuration can be also changed to
by moving default.conf file to Code::Blocks application directory
- create empty Code::Blocks project choosing "GNU GCC Extensa" as compiler in wizard and unchecking "Debug" configuration
- in project properties, "Project settings" tab check "This is a custom Makefile"
- in project properties, in "Build targets" tab rename "Release" target to "all"; duplicate this target twice naming it "clean" and "flash"; you may need to refer later to Makefile for additional targets that would be useful
- in project properties, "C/C++ parser options" add "C:\Espressif\ESP8266_SDK\include" as search path to be able to open SDK files from inside IDE
- add .c, .h files and Makefile to Code::Blocks project (as you prefer, actual build is managed with make)
- replace mkdir with "mkdir.exe" inside Makefile
- specify COM port for programming inside Makefile
- select target ("all" for building, "flash" for building and loading) and build project; pull down GPIO0 and reset board earlier if needed to enter programming mode
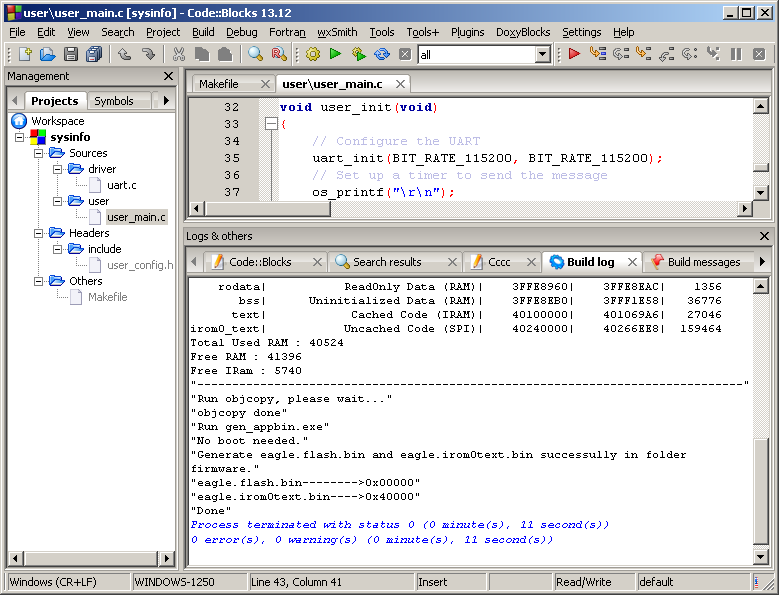
"Generic" Code::Blocks project - with simplest ESP8266 projects from SDK it would be enough to copy it to example project directory: ESP8266.cbp (with more complicated: adding source files to project and adding additional targets if they exist in makefile).
Examples with projects "ported" to Code::Blocks
blinky: toggles LED connected to PORT2
Be aware that there are minor incompatibilities between SDK/DevKit versions that may result in build error when rebuilding with newer DevKit than tested.
Here is "blinky" updated to work with SDK 2.0.9: blinky_sdk_2_0_9.zip.
sysinfo: prints basic OS info
Sysinfo UART output:
BMP180 - very cheap digital atmospheric pressure (and temperature) sensor, successor of BMP085. Working with similar to ESP8266
voltage range (1.8V-3.6V), connected with I2C. DIP-ready modules with MIC5205 voltage regulator and pull-up resistors are priced at ~$1.50.
i2c_bmp180.7z: prints temperature and pressure from BMP180 sensor
i2c_bmp180_ck.7z: same application but with makefile swapped from another project. This makefile
is using esptool-ck for flashing and using additional UART converter lines to control ESP8266 reset and GPIO0 (RTS controls RESET or CH_PD, DTR controls GPIO0) thus allowing automatical switching to bootloader mode when starting programming.
Cheap UART converters based on FT232RL (or quite probably its clone) seem to be good choice for programming interface as they have switchable UART
voltage levels (5V, 3.3V needed for ESP8266, even more with external regulators) and all FTDI pins broken out.
Note: if you have to use 5V UART connect ESP8266 TX directly to UART RX and connect UART TX to EXP8266 using voltage diviver - 2k2 resistor in series and 4k7 to GND seem to do the job.
BMP180 + HTTP client
bmp180_httpclient.7z
Simple application that is reading periodically temperature and atmospheric pressure with BMP180 sensor
and posts it to HTTP server. Example php script that can be used for test purposes (dumping received data with timestamps into text file):
<?php $s=$_POST['s']; $dest='temp.txt'; $fp = fopen($dest, "a", 0); #open for writing, append mode fprintf($fp, "%s %s\r\n", strftime("%Y%m%d %H:%M:%S",time()), $s); fclose($fp); ?>
Configuration (user_config.h) needed: WiFi network name and password, http host and script name. As you can see above passed POST parameter is named "s".
Compiling and linking with another SDK version may require minor changes. Changing from DevKit 2.0.4 to 2.0.6 (that is to SDK 1.1.1) required adding function linked from libmain.a:
void user_rf_pre_init(void) { // system_phy_set_rfoption() can be called from here }bmp180_httpclient_SDK_1_1_1.7z
BMP180 + Si7021 + HTTP client
Yet another iteration of my "web thermometer" project. Definitely cheapest and easiest to assemble so far,
but also easiest to write software for - ESP8266 SDK is easier to setup than e.g. MPLAB+C18 and there are
tons of examples of ESP8266 usage for similar purposes.
Si7021 is a cheap and popular temperature and humidity sensor, compatible with SHT21. Mini-modules are available for under $4:
Schematic:
bmp180_si7021_httpclient.pdf
Source code:
bmp180_si7021_httpclient.7z (tested with Espressif-ESP8266-DevKit-v2.0.6-x86.exe).
bmp180_si7021_httpclient_20151125.zip: fixed invalid string generated for negative temperatures (tested with DevKit 2.0.8)
ADC
ADC pin (TOUT, pin #6) is not directly available on ESP01 module (unlike the ESP07 or ESP12), but with some care
piece of kynar wire can be soldered directly to the chip.
Example is updated to work with DevKit 2.0.8:
- included user_interface.h ("implicit declaration of 'system_adc_read'")
- added to project and included espmissingincludes.h ("implicit declaration of 'ets_sprintf', 'ets_timer_setfn'")
For test purposes I've modified bus_pirate.dll miniscope plugin to capture ADC data continuously from serial port. Quite significant ADC measurement disturbances are visible in resulting plots:
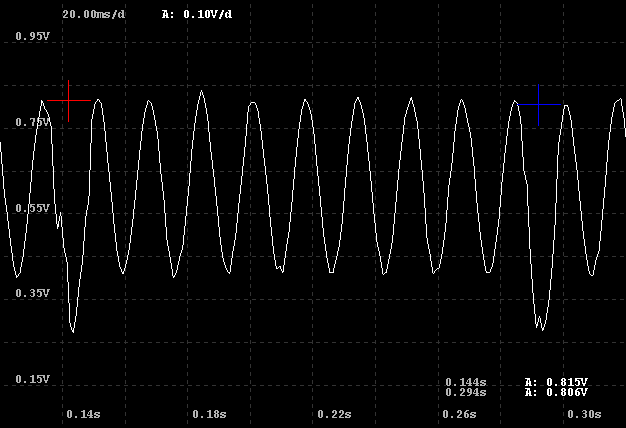
ADC_test_scope.zip
esp8266_dll.zip
See also: VoIP/RTP pager